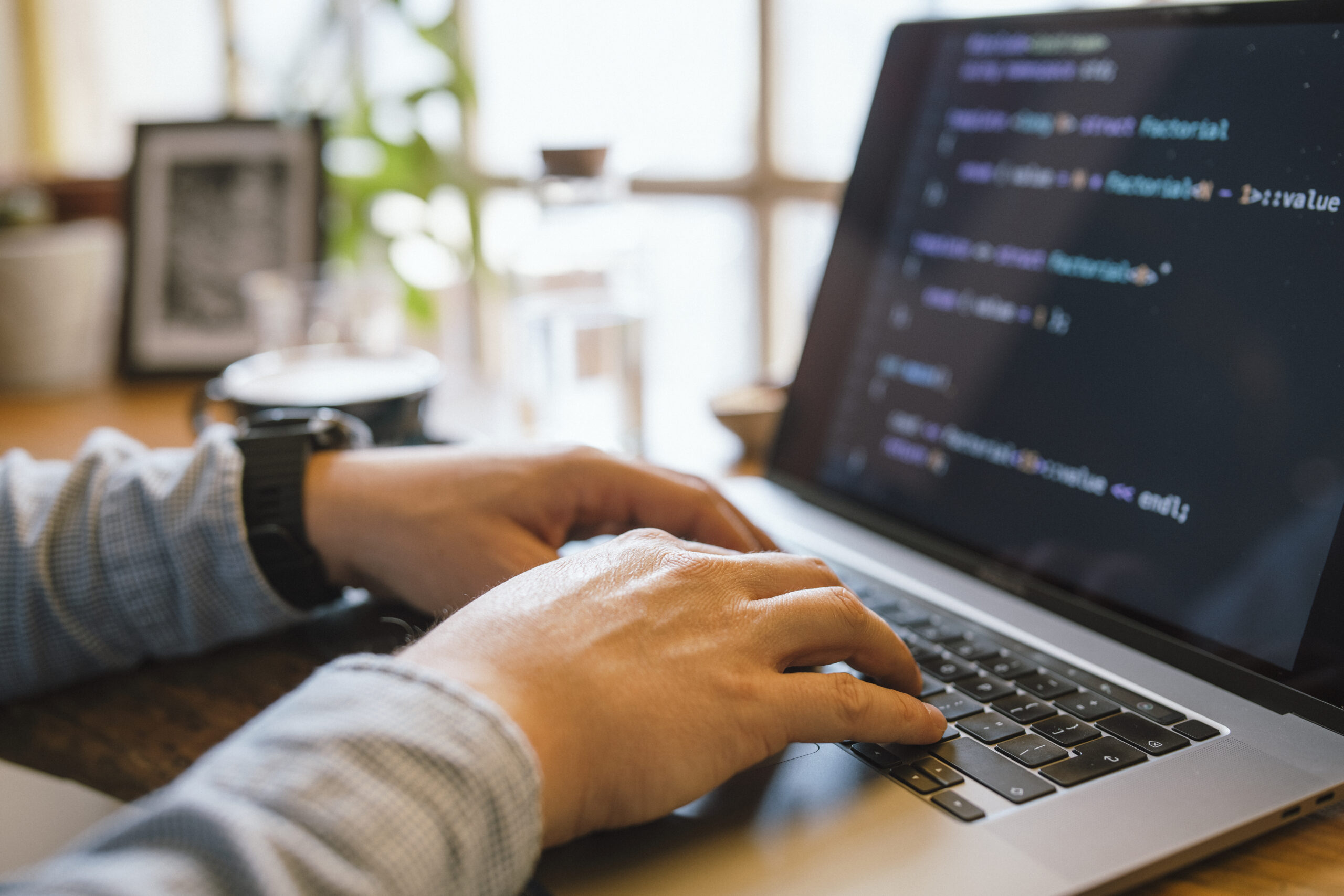
Debugging is Just about the most necessary — however usually neglected — abilities within a developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about understanding how and why items go Improper, and Finding out to Imagine methodically to solve issues successfully. Whether or not you're a novice or maybe a seasoned developer, sharpening your debugging skills can save hours of aggravation and drastically increase your productiveness. Here's quite a few procedures to help builders amount up their debugging activity by me, Gustavo Woltmann.
Learn Your Applications
On the list of fastest strategies builders can elevate their debugging techniques is by mastering the applications they use everyday. When producing code is just one Element of progress, figuring out the way to communicate with it successfully during execution is Similarly significant. Modern day improvement environments occur Outfitted with effective debugging capabilities — but many builders only scratch the surface area of what these tools can perform.
Get, for instance, an Built-in Development Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code within the fly. When utilized the right way, they Allow you to notice just how your code behaves throughout execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer equipment, like Chrome DevTools, are indispensable for entrance-end builders. They permit you to inspect the DOM, watch network requests, watch authentic-time efficiency metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn annoying UI issues into workable duties.
For backend or method-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep Manage about running procedures and memory management. Mastering these resources may have a steeper Finding out curve but pays off when debugging functionality issues, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become at ease with version Regulate programs like Git to understand code heritage, locate the precise second bugs were being introduced, and isolate problematic alterations.
Finally, mastering your resources means heading beyond default settings and shortcuts — it’s about establishing an personal expertise in your improvement setting to make sure that when issues come up, you’re not missing at the hours of darkness. The greater you realize your resources, the more time you are able to invest solving the actual dilemma in lieu of fumbling by the procedure.
Reproduce the condition
One of the more critical — and often overlooked — steps in efficient debugging is reproducing the issue. Just before jumping into the code or creating guesses, developers need to produce a reliable natural environment or circumstance the place the bug reliably appears. Without having reproducibility, fixing a bug becomes a match of possibility, usually leading to squandered time and fragile code alterations.
The first step in reproducing a problem is accumulating just as much context as you can. Request concerns like: What actions triggered The problem? Which atmosphere was it in — development, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you've, the easier it will become to isolate the exact conditions underneath which the bug occurs.
When you finally’ve gathered adequate facts, make an effort to recreate the problem in your local environment. This might mean inputting precisely the same information, simulating very similar user interactions, or mimicking technique states. If the issue appears intermittently, take into consideration composing automated assessments that replicate the edge scenarios or state transitions included. These tests not just assistance expose the trouble and also stop regressions Sooner or later.
Occasionally, The problem may very well be surroundings-certain — it'd happen only on specific functioning methods, browsers, or beneath unique configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the problem isn’t only a phase — it’s a mindset. It involves patience, observation, along with a methodical technique. But as you can consistently recreate the bug, you are presently midway to repairing it. That has a reproducible state of affairs, you can use your debugging resources additional successfully, check probable fixes safely, and communicate a lot more Plainly with the workforce or buyers. It turns an summary criticism right into a concrete obstacle — Which’s the place builders prosper.
Browse and Realize the Mistake Messages
Mistake messages tend to be the most beneficial clues a developer has when something goes Mistaken. Instead of looking at them as aggravating interruptions, builders really should master to take care of mistake messages as immediate communications within the procedure. They often tell you exactly what transpired, where by it transpired, and from time to time even why it took place — if you understand how to interpret them.
Begin by reading through the concept meticulously and in full. Lots of builders, particularly when under time tension, look at the initial line and right away begin earning assumptions. But further while in the error stack or logs may well lie the real root result in. Don’t just copy and paste mistake messages into search engines like google and yahoo — browse and have an understanding of them initially.
Split the error down into pieces. Could it be a syntax error, a runtime exception, or simply a logic error? Does it point to a certain file and line range? What module or purpose induced it? These questions can guideline your investigation and position you toward the liable code.
It’s also valuable to know the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java usually abide by predictable designs, and Understanding to acknowledge these can significantly hasten your debugging system.
Some glitches are vague or generic, As well as in People conditions, it’s vital to look at the context by which the error happened. Check associated log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede more substantial difficulties and supply hints about possible bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Learning to interpret them appropriately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a a lot more successful and self-assured developer.
Use Logging Wisely
Logging is Among the most impressive tools inside a developer’s debugging toolkit. When employed efficiently, it provides true-time insights into how an application behaves, supporting you fully grasp what’s occurring beneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what amount. Prevalent logging concentrations involve DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information during development, Facts for typical gatherings (like thriving get started-ups), Alert for possible problems that don’t break the applying, Mistake for true troubles, and FATAL when the method can’t continue.
Avoid flooding your logs with too much or irrelevant details. Far too much logging can obscure significant messages and slow down your method. Concentrate on essential functions, state changes, enter/output values, and significant selection points in the code.
Format your log messages clearly and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s easier to trace troubles in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all without having halting This system. They’re Specially valuable in generation environments exactly where stepping by website code isn’t achievable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Using a perfectly-believed-out logging technique, you can decrease the time it's going to take to spot troubles, attain deeper visibility into your apps, and Increase the overall maintainability and dependability within your code.
Think Just like a Detective
Debugging is not merely a technical job—it's a sort of investigation. To correctly determine and correct bugs, builders will have to strategy the method just like a detective resolving a secret. This state of mind will help stop working elaborate issues into manageable components and comply with clues logically to uncover the basis bring about.
Get started by gathering evidence. Look at the signs and symptoms of the trouble: mistake messages, incorrect output, or effectiveness challenges. Just like a detective surveys a crime scene, gather as much related details as it is possible to with no leaping to conclusions. Use logs, examination situations, and consumer experiences to piece alongside one another a transparent photo of what’s going on.
Subsequent, kind hypotheses. Request yourself: What can be producing this actions? Have any variations lately been created towards the codebase? Has this problem occurred before less than identical situation? The target should be to slender down options and identify opportunity culprits.
Then, take a look at your theories systematically. Seek to recreate the situation in the controlled setting. In case you suspect a certain operate or element, isolate it and verify if The difficulty persists. Similar to a detective conducting interviews, request your code concerns and Allow the results lead you nearer to the truth.
Fork out near focus to smaller specifics. Bugs usually disguise while in the least predicted spots—just like a missing semicolon, an off-by-1 mistake, or even a race condition. Be extensive and patient, resisting the urge to patch The problem without entirely comprehending it. Non permanent fixes might disguise the real trouble, only for it to resurface afterwards.
Finally, continue to keep notes on Whatever you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term troubles and help Other individuals have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical expertise, solution issues methodically, and develop into more effective at uncovering hidden problems in intricate methods.
Publish Tests
Producing checks is among the most effective strategies to help your debugging abilities and All round progress effectiveness. Checks not only assist catch bugs early but in addition function a security Web that offers you confidence when creating adjustments on your codebase. A very well-analyzed software is much easier to debug mainly because it allows you to pinpoint precisely in which and when a difficulty happens.
Begin with device tests, which concentrate on personal features or modules. These tiny, isolated exams can rapidly reveal regardless of whether a particular piece of logic is Functioning as anticipated. Whenever a test fails, you immediately know where to glimpse, considerably decreasing the time used debugging. Device assessments are Specially valuable for catching regression bugs—concerns that reappear right after previously being preset.
Upcoming, integrate integration tests and end-to-conclusion assessments into your workflow. These aid ensure that various portions of your application function alongside one another efficiently. They’re specifically useful for catching bugs that come about in sophisticated systems with various elements or solutions interacting. If a little something breaks, your assessments can tell you which Element of the pipeline failed and under what problems.
Creating assessments also forces you to Imagine critically about your code. To check a characteristic correctly, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of comprehension naturally sales opportunities to better code construction and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the test fails persistently, you could give attention to correcting the bug and watch your examination go when the issue is settled. This tactic makes certain that the identical bug doesn’t return Sooner or later.
To put it briefly, creating assessments turns debugging from the frustrating guessing match right into a structured and predictable procedure—supporting you capture more bugs, more quickly plus much more reliably.
Take Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, hoping Alternative after Answer. But The most underrated debugging instruments is solely stepping absent. Having breaks allows you reset your intellect, reduce disappointment, and sometimes see The problem from a new viewpoint.
When you're way too near to the code for way too prolonged, cognitive fatigue sets in. You may begin overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. In this state, your Mind results in being fewer economical at trouble-resolving. A brief walk, a coffee crack, or simply switching to a unique process for 10–15 minutes can refresh your focus. Lots of builders report discovering the root of a dilemma when they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, Particularly all through for a longer period debugging periods. Sitting before a display, mentally stuck, is don't just unproductive but in addition draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You might quickly recognize a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute break. Use that time to maneuver all around, extend, or do anything unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, but it surely really brings about faster and simpler debugging in the long run.
In a nutshell, having breaks just isn't an indication of weak spot—it’s a smart tactic. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Each individual Bug
Each bug you come across is a lot more than just A brief setback—It is really an opportunity to expand for a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can educate you anything important if you take some time to mirror and analyze what went Incorrect.
Commence by inquiring on your own a handful of key concerns after the bug is settled: What induced it? Why did it go unnoticed? Could it are caught before with improved tactics like device tests, code reviews, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Construct more powerful coding routines shifting forward.
Documenting bugs can also be a great habit. Keep a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Everything you realized. As time passes, you’ll start to see styles—recurring difficulties or widespread problems—which you can proactively stay away from.
In team environments, sharing what you've acquired from the bug along with your peers is usually In particular highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast know-how-sharing session, aiding Other people steer clear of the very same concern boosts workforce performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. In the end, many of the greatest builders usually are not those who create fantastic code, but people who consistently find out from their issues.
Ultimately, Each individual bug you correct provides a fresh layer towards your skill established. So future time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, more capable developer because of it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — even so the payoff is large. It tends to make you a more successful, confident, and capable developer. The next time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.